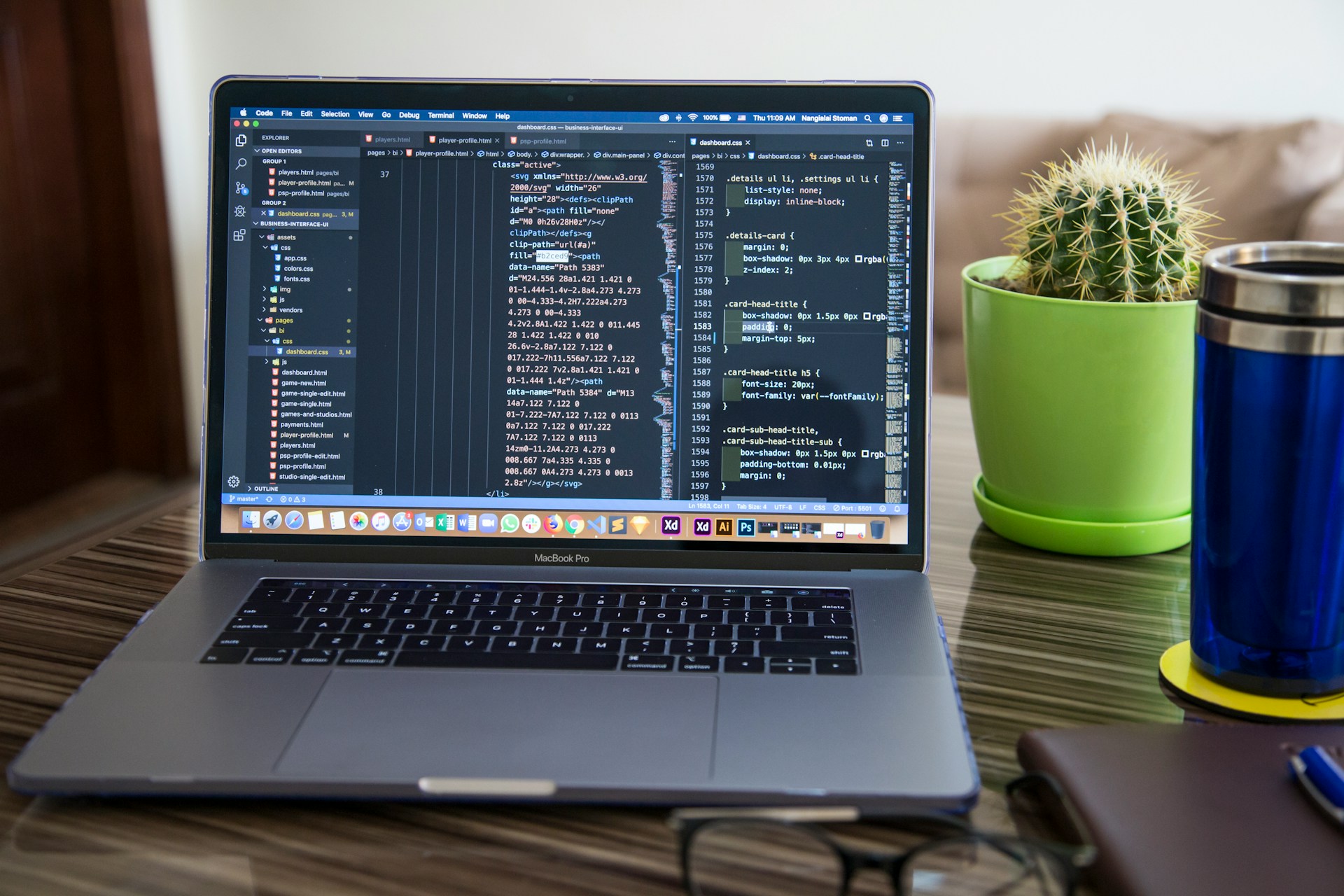
How to Create a Powerful Email Subscription Form and Automate Emails with Google Sheets (No Backend Required!)
In today’s digital world, collecting email addresses is crucial for growing your audience. Whether you’re a blogger, a small business owner, or running a website, having a reliable subscription form is a must. What if I told you that you could build a simple but powerful subscription form, store subscribers in Google Sheets, send thank-you emails automatically, and prevent duplicate sign-ups—all without needing a backend?
In this guide, I’ll walk you through how to:
- Create a simple email subscription form with HTML.
- Integrate the form with Google Sheets to manage your subscribers.
- Automatically send thank-you emails to new subscribers.
- Prevent multiple sign-ups with the same email address.
Step 1: Create the HTML Subscription Form
The first step is to create a basic form where users will input their email address to subscribe. Here’s the HTML for the form:
This form includes:
- An input field where users enter their email.
- A subscribe button to submit the form.
- A hidden container for displaying success or error messages.
Step 2: Set Up Google Sheets as Your Database
We’ll use Google Sheets to store subscriber information. Here’s how to do it:
- Open Google Sheets and create a new spreadsheet.
- Label the first row with headers like: Timestamp, Email, and Email Sent Date.
- Go to Extensions > Apps Script.
In Apps Script, you can write JavaScript to automate email handling and database updates when someone subscribes. Here’s the code you’ll need:
Step 3: Connect the Form to the Apps Script
Now, you need to make the subscription form functional by connecting it to the Apps Script you just created. This can be done with JavaScript and AJAX:
Make sure to replace YOUR_GOOGLE_APPS_SCRIPT_URL
with
the URL of your published Google Apps Script.
Step 4: Prevent Multiple Sign-Ups for the Same Email
This is handled within the Apps Script code above. The script checks whether the email already exists in the Google Sheets database before processing the subscription. If it’s a duplicate, it will return the message "You are already subscribed!" and prevent the email from being added again.
Step 5: Bonus—Send a Personalized Thank You Email
Once someone subscribes, they will automatically receive a thank-you email. This is handled by the MailApp.sendEmail() function in the Apps Script. You can customize the message to match your brand tone.
Conclusion
Now you’ve created a simple yet powerful subscription system that:
- Allows users to subscribe via an email input form.
- Prevents duplicate sign-ups by checking the email in the database (Google Sheets).
- Sends an automatic thank-you email to new subscribers.
This setup is lightweight, easy to maintain, and doesn't require a backend, making it perfect for small to medium-sized websites. By automating this process, you can focus on creating great content while effortlessly building your email list.